Overview
Modulo is a NUS student life application specially designed for busy and motivated NUS students.
As busy students in NUS, we understand that it can be daunting to keep track of various tasks and concurrently do well for exams. Modulo features a one-stop application to keep track of one’s timetable, finances and academic progress. It also includes a quiz feature that facilitates students with actively recalling their study concepts to improve their study outcomes.
The user interacts with Modulo using a CLI, and it features an outstanding user-friendly GUI created with JavaFX. It is written in Java, and has about 35 kLoC.
Summary of contributions
-
Major enhancement: create calendar feature
-
What it does: allows the user to manage their tasks and classes in a weekly calendar view.
-
Justification: This feature improves the product significantly because our targeted user, which are NUS students, needed a fast and convenient way to manage all of their tasks.
-
Highlights: This enhancement affects existing commands and needs to be supported by change of the graphical user interface. The implementation too was challenging as it required changes to existing commands.
-
-
Code contributed: [Functional and test code]
-
Other contributions:
-
Project management:
-
Managed release
v1.3
on GitHub
-
-
Enhancements to existing features:
-
Community:
-
PRs reviewed (with non-trivial review comments): #167
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Calendar
Calendar displays a weekly list of tasks and modules which the user has added. To enter the Calendar section
please enter the command: switch calendar
.
Add a new ToDo Task: add
Adds a new ToDoTask
to the calendar. This ToDoTask
only exist in the week that is currently displayed.
All fields except for TAG
are compulsory fields. You could not add a new task if in the current week there already
exists another task with the same title, day, and deadline.
The fields of ToDoTask
consist of:
- TaskTitle
The name of the task. Prefix: <title>
- TaskDay
The time when the user plan to do the task. Prefix: <day>
.
- TaskDescription
Additional description of the Task
. Prefix: <desc>
.
- TaskDeadline
The date when the task is due in dd-mm-yyyy format. Only valid dates are accepted
(e.g. the date 31-02-2019 does not exist and hence is not accepted). Prefix: <deadline>
.
- TaskTime
Where time when the task occurs, in HH:mm 24-hour format from 00:00 to 23:59. Prefix: <time>
.
- TAG
Tag that can be used to sort the tasks. Prefix: <tag>
.
More than one TAG
can be added to each task, e.g. <tag> High-Priority <tag>Math
Format: add <title>TITLE <day>DAY <desc>DESCRIPTION <deadline>DEADLINE <time>TIME [<tag>TAG]
e.g. add <title>CS2109 Assignment <day>tuesday <desc>Submit code through LumiNUS <deadline>21-10-2019
<time>13:00 <tag>IMPORTANT
If the calendar list gets too long, you can scroll individual days to see the tasks that are not currently on screen. |
Add a new Module Task: addmod
Adds a new ModuleTask
to the calendar. ModuleTask
are the same as ToDoTask
except it doesn’t need a
deadline and it would be automatically added to each week. ModuleTask
is not affected by the command clearweek
.
Format: addmod <title>TITLE <day>DAY <desc>DESCRIPTION <time>TIME [<tag>TAG]
e.g. addmod <title>CS2109 Lecture <day>tuesday <desc>Submit code through LumiNUS <time>13:00
Edit existing task: edit
Edit one or multiple fields of an existing task. INDEX
should be a positive integer and a task with the number INDEX
should already exist on the current week’s calendar. Editing the deadline is only available for ToDoTask
.
Format: edit INDEX <title>TITLE <day>DAY <desc>DESCRIPTION <deadline>DEADLINE <time>TIME <tag>TAG
e.g. edit 1 <title>CS2108 Assignment <day>friday
Delete existing task: delete
Delete an existing task. INDEX
should be a positive integer and a task with the number INDEX
should already exist on the current week’s calendar.
Format: delete INDEX
e.g. delete 1
Listing all tasks : list
Shows a list of all task in the calendar.
Format: list
Sort tasks: sort
Sort the tasks according to the specified SORT_TYPE
.
There are three SORT_TYPE
:
- sort time
will sort the tasks according to the their time in ascending order
- sort deadline
will sort the tasks according to their deadline in ascending order
- sort title
will sort the tasks according to their title in ascending alphabetical order
When the application is launched for the first time, the tasks are sorted according to time
. Subsequently, it will
use the sorting type that is last used in the previous session.
Format: sort SORT_TYPE
e.g. sort deadline
Change week: go
Go to the specified week WEEK_NUMBER
. The WEEK_NUMBER
must between 0 to 14 inclusive. The default week is week 0 and
would be shown every time the application is launched.
Format: go WEEK_NUMBER
e.g. go 2
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Calendar
Calendar week change
Implementation
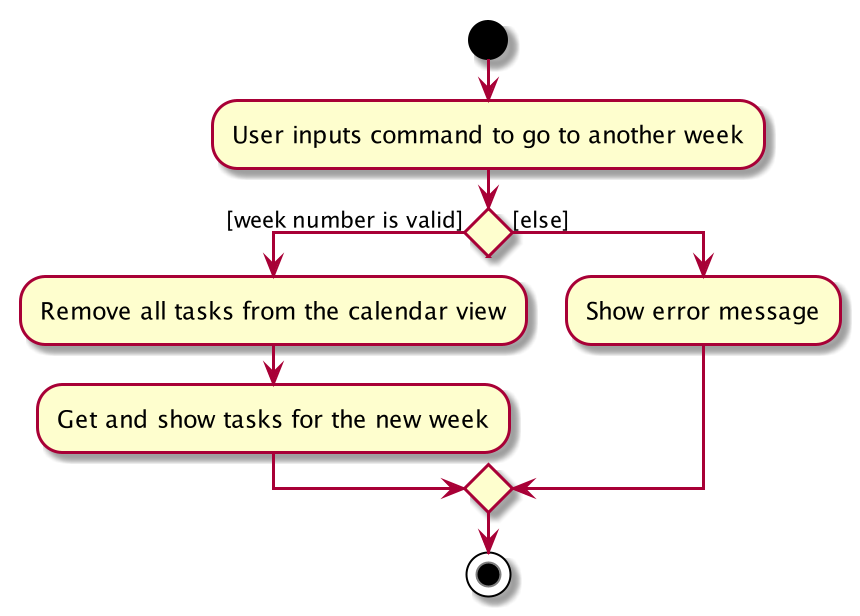
Calendar week change is done by removing all tasks from the calendar and adding all the tasks that either have the new
corresponding week number or is an instance of ModuleTask
.
Design Considerations
Aspect: How go WEEK_NUMBER
executes
-
Alternative 1 (current choice): Save all data in a single json file.
-
Pros: No need to switch storage file.
-
Cons: Unnecessary reading of data from other weeks. Need to add filter to get only the relevant data.
-
-
Alternative 2: Read and load for each week from separate json storage file.
-
Pros: Easy to clear data from a certain week.
-
Cons: Need to switch storage file each time the user go to the next/previous week.
-
Persistent ModuleTask
Implementation
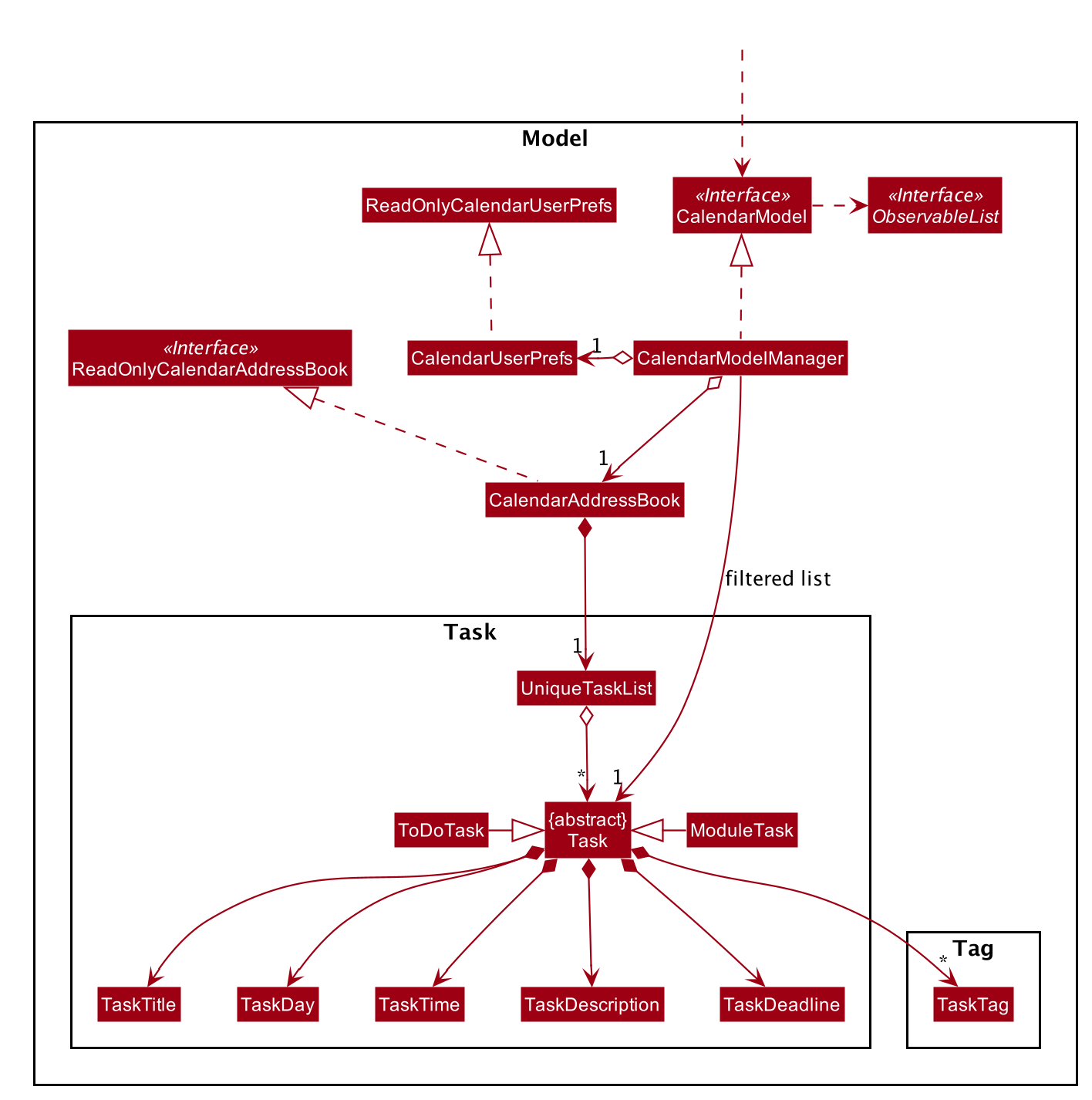
The abstract class Task
has two subclasses: ToDoTask
and ModuleTask
. ToDoTask
only exists in its corresponding
week while ModuleTask
is persistent. When a ModuleTask
is added to any arbitrary week, it would be automatically
added to all of the other weeks and when a certain week is cleared using the clearweek
command, it would not affect
the ModuleTask
. Hence, ModuleTask
can only be deleted by using the command clear
or deleting each ModuleTask
manually using delete
.
Design consideration
-
Alternative 1 (current choice): Create separate classes for
ToDoTask
andModuleTask
that both inherits from the abstract classTask
. User use different commandadd
andaddmod
to create the corresponding subclass.-
Pros: Code looks cleaner. Easier to implement a different UI for each subclass.
-
Cons: More code.
-
-
Alternative 2: Use a non-abstract class
Task
for both ToDos and Modules and add a boolean fieldisPersistent
.-
Pros: No need to add new classes.
-
Cons: User needs to input an extra field
<persist> [TRUE/FALSE]
. It is more difficult to differentiate the type ofTask
when reading the code.
-
Sorting
Implementation
Task
can be sorted according to TaskTitle
, TaskDeadline
, or TaskTime
. Each type of sorting has its own
comparator implemented in a method that would return a sorted FilteredList<Task>
.
Design consideration
-
Alternative 1 (current choice): Use an external comparator.
-
Pros: Easy to switch between different sort type.
-
Cons: Need to call the sort method each time the calendar is updated.
-
-
Alternative 2: Set the
Task
class to implement theComparable
interface.-
Pros: Need to switch the
compareTo()
method of eachTask
each timesort
is called. -
Cons: New/edited task would be automatically placed at the correct order.
-
Other class diagrams
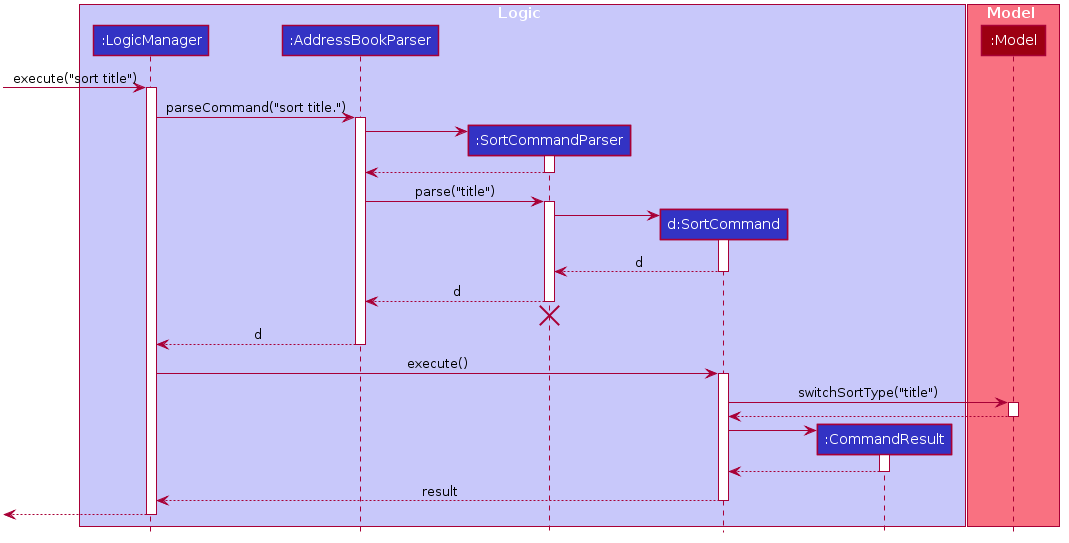
sort title
Command for the feature Calendar